Quick Tip: Capture Geolocation Data in Qualtrics Surveys Using JavaScript
Qualtrics is a widely used experience management platform targeted towards companies and institutions who want to do (statistical) research on, for example, their customers. While Qualtrics can do a lot more, one of its core features is designing and running highly sophisticated surveys.
This particular post is about tracking geolocation data (i.e., the latitude and longitude) from respondents in these surveys. Unfortunately, as of now, the exact (GPS-based) location of a respondent can only be tracked using the Qualtrics Offline App. For all other surveys, for example browser-based ones, the location is only approximated using the IP address and a location database.
Thankfully, Qualtrics supports custom JavaScript for surveys, and therefore we can add GPS-based location tracking relatively easily.
Geolocation Data in Qualtrics Surveys Using JavaScript
In order to track/collect exact - at least more or less - geolocation data, we will rely on both the W3C Geolocation API as well as Qualtrics Embedded Data functionality. The HTML5 geolocation API lets us access the location of the device via the browser, and we can use embedded data fields to add the retrieved data to our survey responses.
In the simplest case, adding GPS-based tracking to a Qualtrics survey is a straightforward two-step process:
First, we need to add additional ‘embedded data’ fields using the Survey Flow tool. Secondly, we will ‘attach’ our custom JavaScript to one of our questions (or the header of the survey). The JavaScript will then get the respondent’s location (after the browser asks for consent), and the location (latitude/longitude) will be stored as embedded data.
The most straightforward implementation might look something like this:
Qualtrics.SurveyEngine.addOnReady(function()
{
// Check Geo. Capabilities
if (!navigator.geolocation){
Qualtrics.SurveyEngine.setEmbeddedData('geo_capability', 'False');
} else {
Qualtrics.SurveyEngine.setEmbeddedData('geo_capability', 'True');
// Retrieve and Save Coordinates
function success(position) {
var lat = position.coords.latitude;
var lng = position.coords.longitude;
Qualtrics.SurveyEngine.setEmbeddedData('geo_lat', lat.toString());
Qualtrics.SurveyEngine.setEmbeddedData('geo_lng', lng.toString());
};
function error() {
console.log('Error')
};
navigator.geolocation.getCurrentPosition(success, error);
}
});
In addition to storing the latitude and longitude (as embedded fields ‘geo_lat’ and ‘geo_lng’ respectively), we are also storing whether the respondent’s device was capable of providing this data (‘geo_capability’). This might be important in order to understand whether our audience is completing the survey on supported devices. Furthermore, while we possibly also would have to mitigate missing consent, we could use this information to provide fallbacks.
Process of adding JavaScript to a question in Qualtrics
Caveats of This Implementation
Of course, this simple implementation is not really suited for larger projects as there is no error handling to speak of, and we do not account for different browsers and devices.
Also, from a software development perspective, we probably would want to encapsulate the functionality a bit more in order to, for example, pull the location multiple times during the survey. We might also want to implement a fallback (e.g., an additional question) if we cannot access the geolocation via the API.
A good starting point for a more production-ready solution could be a library such as Geolocator, which already provides good support for all major browsers and custom fallbacks.
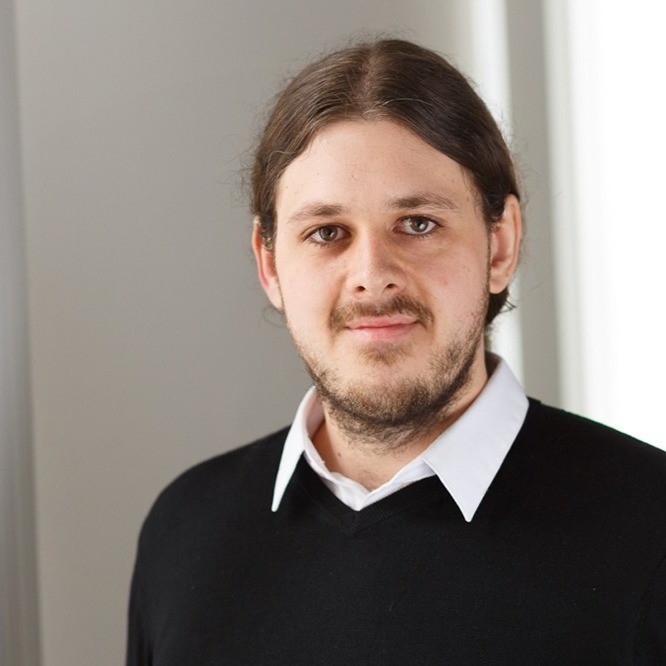
Thank you for visiting!
I hope, you are enjoying the article! I'd love to get in touch! 😀
Follow me on LinkedIn